Well, let me summarize what we understood until now
A] Whatever you see on the browser (including this page), is all pure HTML
B] When the browser "draws" the HTML as buttons, textboxes, colors etc., it also creates a hierarchy of objects called the DOM model.
C] The elements are in this order : HTML --> BODY --> Other Elements
You might be saying "All right, I get it ... but is there a way I can physically see this hierarchy ??". The answer is Yes. But, you should have either Visual Studio or Microsoft Script Editor installed on you machine. Let us see how.
First, create a simple test page called HelloWorld.html. Here's the text
<html> <head> <title>Untitled Page</title> </head> <body> <div id="myDiv"> <input type="button" id="btn" value="Click Me"> </div> </body> </html>
Type the above in a simple notepad and save it as HelloWorld.html. Next, open this page in internet explorer by double clicking on it. You'll see something like this : For debugging purposes, we would have to add some javascript to this page. Let's tweak the HTML slightly :
<html> <head> <title>Untitled Page</title> </head> <body onload="var theDiv = document.getElementById('myDiv');"> <div id="myDiv"> <input type="button" id="btn" value="Click Me"> </div> </body> </html>
As you can see, I have added some javascript to the page (now you know what it looks like). Let's dissect it a little bit before diving into how to debug it. <body onload="XXXXXX" .....
The above line is like effectively telling the browser that "When you start drawing this <body> element, that is, when you "load" this body element, before doing anything else, execute the statements given by XXXXXXX". So, as soon as the browser starts drawing the <body> element on the page, it stops the drawing and first starts executing the javascript. Let's move to the Javascript now. var theDiv = document.getElementById('myDiv'); var -- whenever you create a variable in javascript, the type has to be var only. No matter if it's an int, float, date, even an object, the Type is always VAR. So we declare a variable called "theDiv". Before you wonder what document.getElementById(...) means, let me remind you the hierarchy. HTML --> BODY --> Other Elements
Keep in mind all the page elements (including HTML, and BODY), are part of the page's document. Thats one of the reasons the DOM stands for Document Object Model. So effectively, here's the object hierarchy diagram for any HTML page :
So what document.getElementById('myDiv') is doing is searching for an element called "myDiv" in the document object's children. That is gonna return the <div> element that we created in our HTML, and this value is assigned to the variable theDiv.
Whew, so far so good. Now let's get our hands dirty with some debugging stuff. Open the page in a browser, go to Tools -- > Internet Options --> Advanced --> Browsing section. Uncheck the "Disable Script Debugging (Internet Explorer)" and "Disable Script Debugging (Other)" checkboxes.
Next, go to your page, from menu choose view --> Script Debugger --> Break at next statement
Once done, refresh the page. Immediately you should see the a window similar to the one below :
Choose "Yes", a new session of Visual Studio / Script debugger will open. Once open, you'll see a window like this :
The yellow arrow on the left tells you on which line the debugger is currently at. --Select the text "document.getElementById('myDiv') as shown above. --Right click on the text and choose "Add Watch". The add watch command is used to add the chosen expression to a Watch window, where you can actually see what it's value is. As the program runs, you can see the value change on the fly. On choosing "Add Watch", you'll see a window like this (it usually pops up at the bottom of the Visual Script Debugger window) :
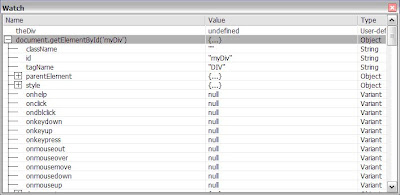
Let's make sense of some of the more important properties -->
-- id : The id of the element (in our case, "myDiv")
-- tagName : The TYPE of the element, whether it's a <div> or an <input> element, or is it <document>, or <body> ... get the point ?!!
-- parentElement : The parent of the current element. In our case, myDiv is a child of the <body> element object (remember the hierarchy ?). So the parentElement should be the document. To confirm, expand the parentElement by clicking the [+] sign, and check out the tagName property of the parent Element. Sure enough, it says "BODY".
Before going further, scroll down to the "document" element and expand it. Here's what you see :
This is the page's document object, which houses all other page element object (including <html>, <body> etc. If you look carefully, you'll see it also contains Scripts, images, links, frames etc. page elements. More on this later.
Next up, OffsetLeft, offsetTop, offsetWidth, offsetHeight : Determine the position of the element on the page.
InnerHTML : The html inside this element
InnerText : If you wanna write some text in an element, you use this.
.
.
.
.
There's a hundred other properties that I'd like to go through, but let's stop here for lack of space and time. For more info, you can always checkout the description for <div> element on the MSDN site http://msdn2.microsoft.com/en-us/library/ms535240.aspx.
To cut a long story short, if you want to look at the internals of the various objects on an HTML page, you can do so by starting to debug the page and looking at various page objects. In my next post, I'll look at the internals of some of the most common operations that you need to perform in javascript while creating websites.
1 comment:
For real, powerful debugging, you'll need to use Firefox, and the amazing Firebug Extension.
Any web developer not using this combo, is considered by the industry to _not_ be a professional web developer.
Post a Comment